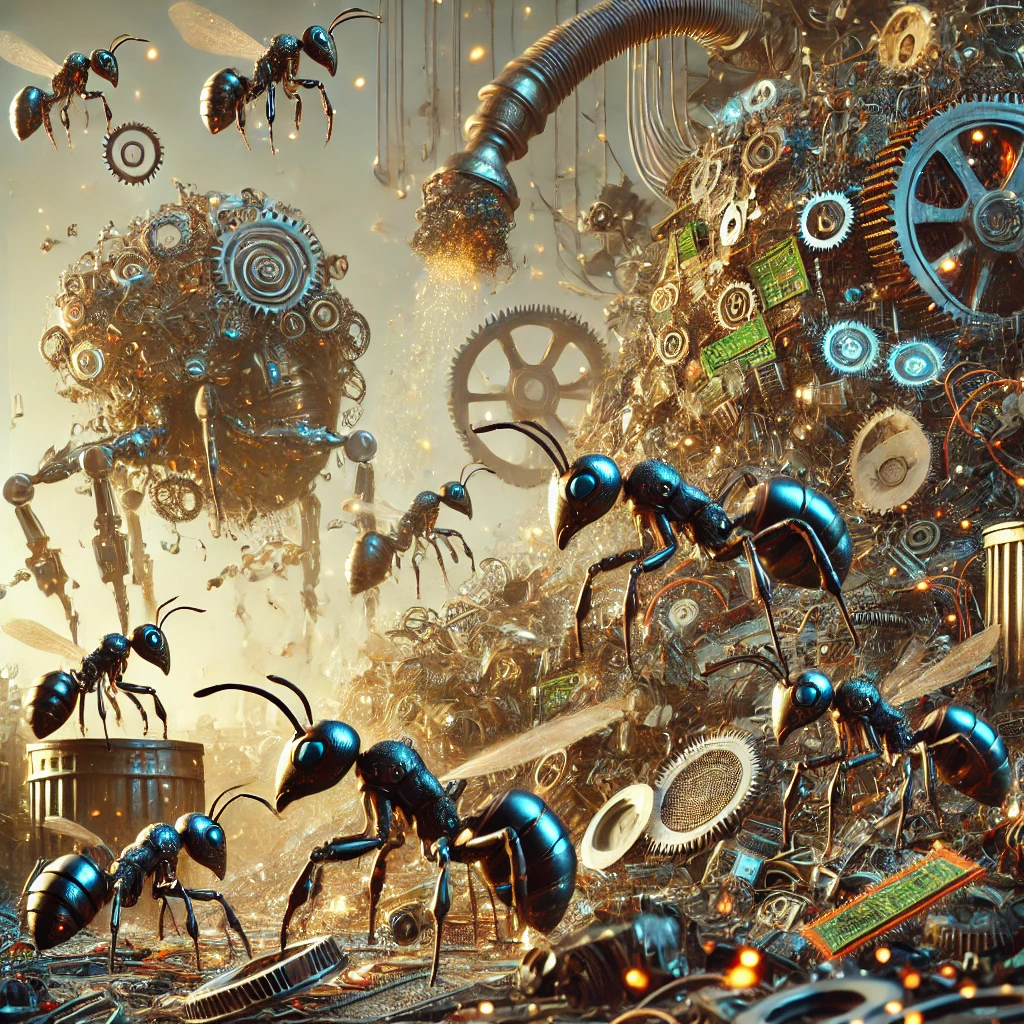
robotic ants and bees are vacuuming up scrap metal and electronic junk
To achieve a swarm intelligent hive simulation:
- Decentralized Control β Workers autonomously decide task assignments.
- Stigmergy (Shared Knowledge) β Workers leave “digital pheromones” (metadata logs) to influence others.
- Colony Optimization β Workers prioritize high-demand tasks based on feedback loops.
- Worker Specialization β Drones self-optimize to become “specialists” in tasks they perform often.
- Queen Coordination β The Queen AI oversees global trends but does not micromanage.
1οΈβ£ Ant Colony Optimization (ACO) π
How it Works
- Workers leave digital pheromones on completed tasks (a database record or memory cache).
- Other workers choose tasks based on the strongest pheromone trails.
- Pheromone decay over time ensures old information does not mislead the hive.
- The Queen AI adjusts global pheromone strength for optimizing task allocation.
π Implementation Each worker logs task success rates and time-to-complete in a shared Redis cache:
import redis
r = redis.Redis(host="10.10.0.9", port=6379, decode_responses=True)
def leave_pheromone(task_id, success_rate):
"""Workers leave digital pheromones based on task success"""
r.zincrby("pheromone_trails", success_rate, task_id)
def choose_task():
"""Workers choose tasks based on strongest pheromone trails"""
task = r.zrevrange("pheromone_trails", 0, 0) # Pick highest-ranked task
return task[0] if task else None
β Result: Workers naturally specialize in tasks they excel at, and frequently needed tasks get prioritized.
2οΈβ£ Particle Swarm Optimization (PSO) π
How it Works
- Each worker AI is a particle in a swarm.
- Particles adjust behavior based on:
- Personal Best Performance (Worker tracks its past efficiency on tasks).
- Global Best Performance (Queen AI tells workers which task solutions are performing best overall).
- Workers share knowledge dynamically and adjust strategies.
π Implementation Each worker AI evaluates its own task efficiency and compares with global best from Queen AI.
def update_worker_behavior(worker_id, task_success_rate):
"""Each worker adapts based on personal & global performance"""
personal_best = r.get(f"worker:{worker_id}:best") or 0
global_best = r.get("global_best") or 0
# If this worker did better than its past performance, update personal best
if task_success_rate > float(personal_best):
r.set(f"worker:{worker_id}:best", task_success_rate)
# If this is better than any other worker, update global best
if task_success_rate > float(global_best):
r.set("global_best", task_success_rate)
β Result: Workers learn over time which task strategies work best and converge toward global optimal behavior.
3οΈβ£ Stochastic Diffusion Search (SDS) π
How it Works
- Instead of all workers processing separate tasks, workers communicate.
- If a worker finds a good solution, it tells other workers to replicate or refine it.
- If a worker fails at a task, it asks neighboring workers for guidance.
π Implementation Workers randomly check if their peers have found a better way to complete a task:
import random
def consult_neighbors(worker_id, task_id):
"""Workers communicate with each other to find better strategies"""
neighbors = ["worker1", "worker2", "worker3"] # Example neighbors
chosen_neighbor = random.choice(neighbors)
best_known_method = r.get(f"worker:{chosen_neighbor}:task:{task_id}")
if best_known_method:
return best_known_method # Worker adopts the better method
return None
β Result: Workers dynamically learn from each other, allowing rapid knowledge transfer.
4οΈβ£ Bee Colony Algorithm π
How it Works
- Workers are divided into Explorers and Foragers:
- Explorers try new tasks and see if they are efficient.
- Foragers do proven tasks that maximize efficiency.
- If an Explorer finds a good task, more Foragers will switch to it.
π Implementation Each worker has a chance to explore or exploit:
import random
def decide_role():
"""Workers randomly decide if they should explore or exploit"""
return "Explorer" if random.random() < 0.3 else "Forager"
β Result: Workers balance exploration of new AI models vs. exploiting well-performing ones.
π Implementing the Hive-Mind in Your Cluster
1οΈβ£ Modify Task Assignment in Node9 (Coordinator)
Modify task routing so workers self-select their tasks instead of blindly accepting them.
π Enhanced Task Routing
def distribute_task(question):
"""Workers self-select tasks based on pheromone strength"""
best_task = choose_task() # Selects task using Ant Colony Optimization
if best_task:
worker_node = get_least_busy_worker()
channel.basic_publish(exchange="", routing_key="task_queue", body=json.dumps({"question": best_task, "worker": worker_node}))
print(f"[Coordinator] Assigned best-fit task to worker {worker_node}")
else:
# No optimized task found, send to Queen AI
channel.basic_publish(exchange="", routing_key="queen_queue", body=json.dumps({"question": question}))
print("[Coordinator] Assigned to Queen AI.")
β Result: Tasks are dynamically chosen by worker AI preference, not just brute-force assignment.
2οΈβ£ Implement Worker Specialization (Adaptive AI Roles)
Each worker tracks its best task and requests similar work.
π Worker Self-Specialization
def request_task(worker_id):
"""Workers request tasks they are good at"""
best_task = r.get(f"worker:{worker_id}:best_task")
if best_task:
return best_task
return choose_task() # Fallback to colony-wide best task
β Result: AI workers develop specialties, just like in real hives.
π₯ Final Architecture
- Node1 & Node2 (Queen AI) β DeepSeek-R1:8B for high-level reasoning.
- Nodes 1, 2, 3 (Workers) β Small AI models for distributed inference.
- Worker AI uses Swarm Intelligence:
- π Ant Colony Optimization for task selection.
- π Particle Swarm Optimization for dynamic learning.
- π Stochastic Diffusion Search for knowledge transfer.
- π Bee Algorithm for balancing exploration/exploitation.