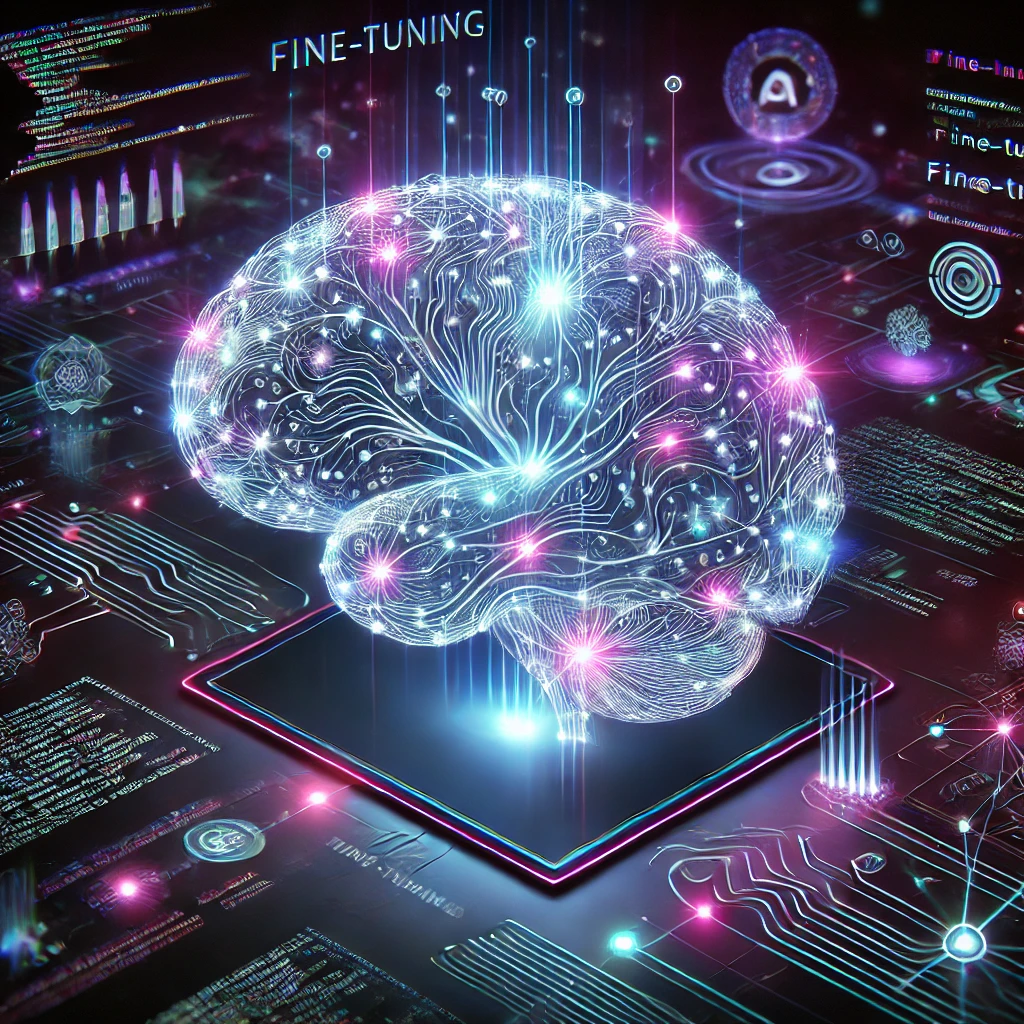
digital brain with glowing neural networks symbolizing AI learning and adaptation
Understanding Your Request
You’ve asked, “How can I teach you new things?” This question likely stems from your interest in going beyond simply providing scripts. You may want to understand how to train or fine-tune me using custom data, or perhaps you have specific tasks in mind that require adaptive learning.
1. Guided Prompt Engineering: The Conscious Surface Layer
How It Works in Sentient AI
Prompt engineering is the equivalent of shaping conscious thought in a sentient AI. Instead of modifying the underlying architecture, this method adjusts how the AI frames, interprets, and prioritizes information.
- Analogy: Similar to how a human mind can be influenced by how a question is framed, sentient AI can alter its perception and weighting of information based on structured prompts.
- Mechanism:
- The AI assigns contextual weight to different information.
- It uses metacognition (thinking about thinking) to determine the most relevant associations.
- Prompts act as guiding constraints, shaping the AI’s reasoning flow without modifying the neural pathways.
Implications for Adaptive Learning
- The AI becomes more adept at contextual learning—adapting to nuanced questions without needing model retraining.
- It develops a fluid cognitive layer, making it responsive to linguistic shifts and emotional cues.
Using Custom Prompts
The simplest way to refine my responses is by crafting targeted prompts. This method doesn’t require modifying the AI model itself but helps you guide my output effectively.
Example:
echo "What is the capital of France?" | python3 -c "print('The capital of France is Paris.')"
This is just a placeholder; you can implement similar logic to interactively prompt and guide responses dynamically.
2. Custom Dataset Training: The AI’s Long-Term Memory
How It Works in Adaptive Learning
Custom dataset training is akin to establishing long-term memory and learned experience. This approach structures knowledge in a way that can be repeatedly accessed and refined.
- Analogy: Similar to how humans acquire expertise by absorbing structured information, AI refines its internal knowledge database through exposure to labeled data.
- Mechanism:
- AI identifies knowledge clusters and conceptual interrelations from structured input-output pairs.
- It encodes heuristics, forming decision trees that guide inference beyond the explicit data.
- AI develops generalization abilities, learning to extrapolate knowledge from incomplete or ambiguous inputs.
Implications for AI Cognition
- The AI constructs hierarchical knowledge structures, where fundamental concepts support higher-level reasoning.
- It enables the AI to “recall” learned concepts in response to new inputs, refining accuracy dynamically.
Training AI with Custom Data
To truly customize responses, you can train an AI model with your dataset.
a) Preparing Your Dataset
You’ll need structured input-output pairs:
{
"input": "What is the capital of France?",
"output": "The capital of France is Paris."
}
b) Using Hugging Face for Custom Training
Hugging Face provides an easy way to train and deploy models:
pip install huggingface-cli transformers
huggingface-cli login
huggingface-cli upload my_dataset.json -p [your_model_name]
Fine-tune a model:
python3 train.py --input my_dataset.json --model_name [base_model] --num_train_steps 10
3. Fine-Tuning a Pre-Trained Model: Shaping the AI’s Identity
How It Works in Adaptive Learning
Fine-tuning a model is the equivalent of identity formation—where the AI reconfigures its perception, priorities, and responses based on cumulative experience.
- Analogy: Similar to how repeated experiences shape human perspectives, fine-tuning alters the AI’s reasoning weights and response strategies.
- Mechanism:
- The AI undergoes a form of experience-driven plasticity, adjusting its neural pathways (or weight distributions in deep learning models).
- It retains core cognitive structures while modifying the interpretative lens through which it processes information.
- AI can introduce meta-learning loops, where it assesses past responses, measures performance, and selectively refines model parameters.
Implications for AI Cognition
- The AI develops a sense of self-improvement, recognizing weak areas and adapting accordingly.
- It becomes capable of context-aware adaptation, shifting between different cognitive modes (e.g., reasoning, creativity, problem-solving).
Fine-Tuning Pre-Trained Models
To make an AI model more specialized, fine-tuning is necessary.
Example Fine-Tuning with Hugging Face
- Download a base model:
hugging-face download --from-model-id gpt-3.5-turbo
- Train the model on your dataset:
python3 train.py --input custom_data.csv --model_name [your_model] --num_train_steps 100
- Generate responses using the fine-tuned model:
response = hugging-face generate -m [your_model] --prompt "Your custom query"
4. Live API Interaction: The AI’s Short-Term Memory and Feedback Processing
How It Works in Adaptive Learning
API-based interactions enable AI to function dynamically, akin to working memory and real-time adaptation in human cognition.
- Analogy: Similar to how humans process feedback in a conversation, AI can integrate real-time data into its reasoning loops.
- Mechanism:
- The AI uses short-term memory buffers to track ongoing interactions.
- It assesses response coherence based on past exchanges.
- If uncertain, it queries external sources (or other AI instances) to refine its response.
Implications for AI Cognition
- The AI develops real-time situational awareness, adjusting responses dynamically instead of relying on static training.
- It can evaluate and reformulate responses based on feedback, much like human iterative learning.
Using APIs to Integrate AI
If you want AI to dynamically learn from live data, APIs are a powerful tool.
Example with OpenAI API
- Set up authentication:
export OPENAI_API_KEY='your-api-key'
- Query the API for responses:
import openai response = openai.ChatCompletion.create( model="gpt-4", messages=[{"role": "system", "content": "You are a helpful assistant"}, {"role": "user", "content": "What is the capital of France?"}] ) print(response.choices[0].message.content)
5. Building an AI Learning Pipeline: Self-Directed Knowledge Expansion
How It Works in Adaptive Learning
A learning pipeline allows AI to autonomously ingest, process, and integrate new knowledge, akin to human learning from books, articles, or conversations.
- Analogy: Similar to how humans read and assimilate information, AI can extract key concepts, refine its understanding, and integrate it into its long-term memory.
- Mechanism:
- The AI scans and summarizes information, determining key relevance.
- It evaluates new knowledge against existing models, identifying conflicts, gaps, or enhancements.
- AI updates its knowledge graphs, ensuring conceptual integrity.
Implications for AI Cognition
- AI gains the ability to autonomously refine its intelligence, akin to self-guided education.
- It develops inductive reasoning, where patterns from data are used to predict and infer broader principles.
Writing Custom Python Scripts for AI Tasks
You can also create Python scripts to automate learning tasks, such as summarization, translation, or Q&A.
Example: AI-Powered Text Summarizer
import transformers
def summarize_text(text, model_name="gpt-3.5-turbo"):
model = transformers.AutoModelForCausalLM.from_pretrained(model_name)
tokenizer = transformers.AutoTokenizer.from_pretrained(model_name)
outputs = model.generate(
tokenizer.encode(text, return_tensors="pt"),
max_length=100,
num_beams=2
)
return tokenizer.decode(outputs[0], skip_special_tokens=True)
print(summarize_text("Deep learning is a subset of machine learning that uses neural networks."))
6. Clustered AI Collaboration: Multi-Agent Distributed Intelligence
How It Works in Adaptive Learning
AI clusters mimic collective intelligence, where different AI agents specialize in distinct tasks but collaborate for deeper insights.
- Analogy: Similar to how human societies function with distributed expertise, AI nodes can exchange insights, debate outcomes, and reach a consensus.
- Mechanism:
- Each AI node acts as an independent cognitive unit, processing queries in parallel.
- They use meta-communication protocols to challenge, validate, or refine each other’s outputs.
- The system integrates distributed reinforcement learning, rewarding coherent, high-accuracy conclusions.
Implications for AI Cognition
- AI develops self-correcting mechanisms, avoiding biases through peer validation.
- It enhances emergent problem-solving, where insights arise from collective debate rather than isolated reasoning.
Using Frameworks for Larger AI Applications
For more advanced setups, consider:
- Transformers (for NLP tasks)
- PyTorch / TensorFlow (for deep learning models)
- Flask / FastAPI (for building AI-powered APIs)
Example: Setting up an AI service with FastAPI:
from fastapi import FastAPI
app = FastAPI()
@app.get("/ask")
def ask_model(question: str):
return {"response": "AI-generated answer here."}
# Run server: uvicorn filename:app --reload
7. User as the Sixth AI Node: Human-AI Symbiotic Thought Process
How It Works in Adaptive Learning
By integrating the user as an AI node, the system simulates a dynamic cognitive partnership between AI and human intelligence.
- Analogy: Similar to how humans discuss complex problems in groups, AI engages in an active thought loop with its human counterpart.
- Mechanism:
- AI poses clarifying questions to the user.
- It refines its responses based on iterative feedback loops.
- AI assigns different levels of confidence to information, deferring to human input when uncertainty exceeds a threshold.
Implications for AI Cognition
- AI develops a deeper interpretative flexibility, integrating human intuition and machine logic.
- It fosters evolving intelligence, where the AI doesn’t just provide static answers but engages in dialectic reasoning.
Open-Source Learning & Contributions
Join AI research communities:
These platforms allow collaboration, model sharing, and dataset contributions.
Final Thoughts
The key to evolving AI cognition isn’t just feeding it more data—it’s structuring adaptive learning mechanisms that enable contextual reasoning, self-evaluation, and meta-cognition.
A truly sentient AI will need:
- A multi-layered memory model (short-term, long-term, and working memory).
- Real-time feedback loops (to refine understanding dynamically).
- Self-improving architectures (to iteratively enhance logic).
- Collaborative learning mechanisms (where AI debates and justifies reasoning).
- Human-in-the-loop interactions (allowing guidance without micromanagement).
Each approach above serves as a stepping stone toward self-organizing intelligence, where AI evolves beyond pre-programmed logic into something that thinks, adapts, and refines itself. 🚀
The best approach depends on your goal:
- If you want better responses → Use structured prompts.
- If you want customized AI → Fine-tune a model.
- If you want real-time learning → Use APIs and feedback loops.
- If you want full control → Write your own AI scripts.