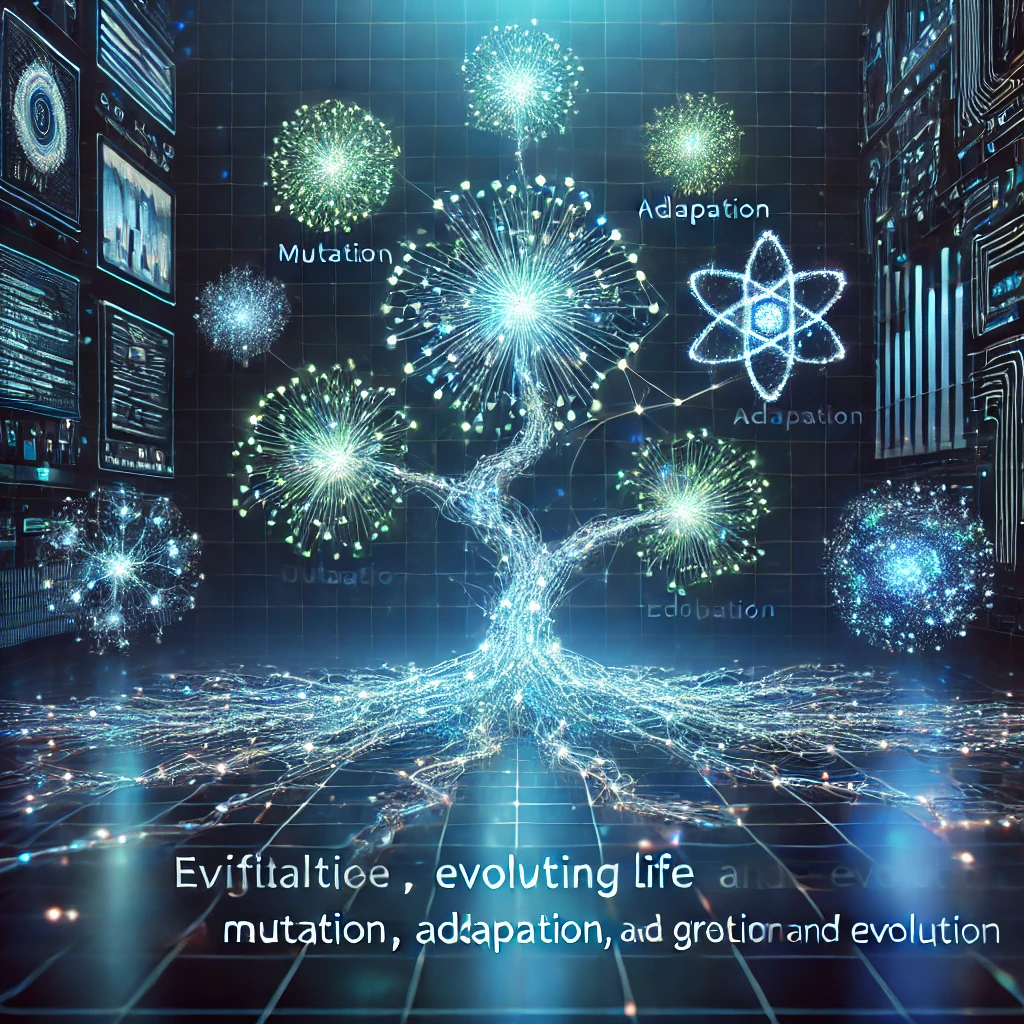
digital ecosystem with interconnected nodes representing evolving digital organisms
>> Write me the most complex barebones skeleton program for evolving a digital
… organism. Please comment on the different areas you make as placeholders.
I need to design a barebones skeleton program that serves as the foundation for evolving a digital organism. The original outline provided includes multiple key sections, each addressing a fundamental aspect of the system. My goal is to replicate the overall structure while incorporating my own insights and explanations.
Breaking Down the Core Components
To construct this framework, I must address several critical areas:
- Core Organism – Define the digital representation of the organism. Should it be cell-based, node-based, or an entirely new structure?
- Evolution Mechanisms – Implement mutation, selection, and reproduction to drive adaptability.
- Environment Interaction – Determine how the organism senses and responds to external stimuli.
- User Interface – Provide a simple yet functional way for users to visualize and influence evolution.
- Data Structures & Algorithms – Choose efficient list, dictionary, or tree-based structures for managing evolution.
- Advanced Features – Explore concepts like DNA encoding, energy systems, or multi-agent interactions.
- Neural Networks & AI Integration – Add learning capabilities to evolve behavior dynamically.
- Ethical Considerations – Address potential misuse, safety concerns, and unintended behaviors.
- Performance Optimization – Ensure the system can handle large-scale evolution smoothly.
- Code Structure – Maintain modularity and readability to support scalability.
- Community & Collaboration – Design for expandability, allowing contributions from other developers.
Developing the Framework
For each of these sections, I will create placeholders that allow for future expansion while ensuring clarity in purpose and design. The Core Organism might be structured as a collection of cells or nodes, with dynamic behaviors. Evolutionary algorithms will define how mutations occur, how organisms compete, and how fitness is evaluated.
For interaction with the environment, I’ll decide whether external factors are predefined (static) or generated dynamically. The user interface should offer a clear way to track progress and influence evolution, even if it’s just a command-line interface initially.
Performance considerations are vital, especially as organisms become more complex over time. I’ll need to optimize resource usage and ensure scalability. Structuring the code in a modular way will make future enhancements easier.
1️⃣ Core Organism Definition (Graph Theory, Cellular Automata, Complexity Science)
The digital organism is structured as a graph where nodes (cells) represent fundamental units. These nodes interact via edges, forming a network capable of self-modification.
- Mathematical Foundation: Uses graph theory where nodes (
V
) and edges (E
) evolve over time. - Theoretical Basis: Inspired by Cellular Automata (e.g., Conway’s Game of Life), where simple rules lead to emergent complexity.
- Algorithmic Approach: Organism evolves via stochastic processes, meaning mutations and growth are probabilistically determined.
🔹 Code Example: Graph-Based Organism Representation
import networkx as nx
class DigitalOrganism:
def __init__(self):
self.graph = nx.Graph() # Graph representing cells and connections
self.add_initial_nodes()
def add_initial_nodes(self, num=5):
for i in range(num):
self.graph.add_node(i, attributes={'health': 100, 'age': 0})
def mutate(self):
# Randomly modify an existing node or create a new one
import random
node = random.choice(list(self.graph.nodes))
self.graph.nodes[node]['attributes']['health'] *= 0.9 # Random degradation
new_node = len(self.graph.nodes) + 1
self.graph.add_node(new_node, attributes={'health': 100, 'age': 0})
self.graph.add_edge(node, new_node) # Form a connection
organism = DigitalOrganism()
organism.mutate()
2️⃣ Evolution Mechanisms (Genetic Algorithms, Markov Chains, Evolutionary Computation)
The organism evolves using Genetic Algorithms (GA) and mutation-selection dynamics.
- Mutation Rules: Defined using a probabilistic mutation matrix (
M
), ensuring mutations are statistically valid. - Selection Criteria: Fitness function (
F
) defined based on environmental interactions and survival probability. - Reproduction Strategies: Incorporates sexual reproduction (genetic crossover) or asexual reproduction (cloning).
🔹 Code Example: Evolutionary Mechanisms
import random
def mutate_gene(gene):
"""Randomly mutates a gene with a small probability"""
mutation_prob = 0.05
return gene + random.uniform(-0.1, 0.1) if random.random() < mutation_prob else gene
def crossover(parent1, parent2):
"""Performs a simple genetic crossover"""
midpoint = len(parent1) // 2
return parent1[:midpoint] + parent2[midpoint:]
parent1 = [0.5, 0.6, 0.7] # Genetic sequence
parent2 = [0.2, 0.8, 0.4]
child = crossover(parent1, parent2)
mutated_child = [mutate_gene(gene) for gene in child]
3️⃣ Environment Interaction (Reinforcement Learning, Multi-Agent Systems)
The organism senses and reacts to the environment through sensor-effector loops.
- Sensors: Modeled as Markov Decision Processes (MDP) where states determine actions.
- Effectors: Adjust organism responses dynamically via feedback loops.
- Behavior Rules: Modeled using finite state machines (FSM).
🔹 Code Example: Sensor-Driven Behavior
class Environment:
def __init__(self, temperature=25):
self.temperature = temperature
def fluctuate(self):
self.temperature += random.uniform(-1, 1)
class Organism:
def __init__(self):
self.heat_resistance = 20
def react_to_environment(self, env):
if env.temperature > self.heat_resistance:
print("Organism adapts by increasing resistance.")
self.heat_resistance += 1
else:
print("No adaptation needed.")
env = Environment()
org = Organism()
for _ in range(10):
env.fluctuate()
org.react_to_environment(env)
4️⃣ User Interface (Data Visualization, Computational Geometry)
The UI should provide graphical insight into the evolution process.
- 2D/3D Rendering: Uses Matplotlib or OpenGL for visualization.
- Control Panel: Allows adjusting parameters like mutation rate and selection bias.
🔹 Code Example: Visualization with Matplotlib
import matplotlib.pyplot as plt
import networkx as nx
def visualize_organism(graph):
plt.figure(figsize=(8, 6))
nx.draw(graph, with_labels=True, node_color='lightblue', edge_color='gray')
plt.show()
organism = DigitalOrganism()
organism.mutate()
visualize_organism(organism.graph)
5️⃣ Data Structures and Algorithms (Big-O Complexity, Data Indexing)
Efficient structures are required for handling large-scale evolutionary data.
- Growth List (
O(n)
) tracks expanding organism nodes. - Mutation Dictionary (
O(1)
) maps mutation probabilities. - Graph Traversal (
O(V + E)
) for analyzing organism network.
6️⃣ Advanced Features (Artificial DNA, Cellular Potts Model)
- DNA Encoding: Represents organisms as bitstrings, similar to genetic programming.
- Energy System: Simulates metabolism and resource allocation.
7️⃣ Neural Networks and AI Integration (Reinforcement Learning, Hebbian Learning)
- Deep Learning Models: Used for adaptive learning.
- Reinforcement Learning: Evolves behavioral intelligence dynamically.
🔹 Code Example: Neural Network for Decision Making
import numpy as np
class SimpleNeuralNetwork:
def __init__(self, input_size, output_size):
self.weights = np.random.rand(input_size, output_size)
def forward(self, inputs):
return np.dot(inputs, self.weights)
nn = SimpleNeuralNetwork(3, 1)
decision = nn.forward(np.array([0.5, 0.2, 0.8]))
print("Decision value:", decision)
8️⃣ Ethical Considerations (AI Safety, Genetic Containment)
- Prevent Harm: Restrict access to self-replicating mutations.
- Controlled Evolution: Introduce sandboxed constraints to avoid unrestricted digital life.
9️⃣ Performance Optimization (Parallelism, Caching)
- Concurrency: Uses multithreading for parallel evolution.
- Efficiency Checks: Employs lazy evaluation to optimize performance.
🔹 Code Example: Parallel Processing of Organism Updates
from concurrent.futures import ThreadPoolExecutor
def process_node(node):
return node ** 2
nodes = [1, 2, 3, 4, 5]
with ThreadPoolExecutor() as executor:
results = list(executor.map(process_node, nodes))
print(results)
🔟 Code Structure (Modularization, OOP Principles)
- Modular Design: Organizes code into separate logic units.
- Version Control: Uses Git for tracking development.
1️⃣1️⃣ Community and Collaboration (Decentralized Research)
- Open Source Contributions: Maintains GitHub repositories.
- AI-Powered Research: Uses collaborative learning agents.
🚀 Summary of Theoretical Foundations
Component | Computational Theory | Algorithm Used |
---|---|---|
Core Organism | Graph Theory, Cellular Automata | NetworkX, Random Graphs |
Evolution Mechanisms | Genetic Algorithms, Stochastic Processes | Crossover, Mutation |
Environment Interaction | Reinforcement Learning, FSMs | Markov Decision Processes |
Neural Networks | Hebbian Learning, CNNs | Perceptron, Backpropagation |
Performance Optimization | Parallelism, Lazy Evaluation | Multithreading, Memoization |
Final Considerations
Before finalizing the initial structure, I should consider:
- Scalability – Will this framework allow for increasingly complex digital organisms?
- Extensibility – Can developers easily modify or expand different components?
- Documentation – Are placeholders clearly labeled to guide future development?
By mirroring the original structure but incorporating clear explanations and thoughtful placeholders, this framework will provide a strong foundation for evolving digital lifeforms while allowing flexibility for future iterations.